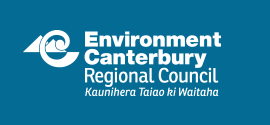
Water Abstraction API
On this page
Introduction
Terminology
Workflow
Endpoints
Frequently Asked Questions
Roadmap
Support
Introduction
This Application Programming Interface (API) allows service providers to submit water abstraction data to Environment Canterbury. The endpoints enable the creation and management of metering points, and the submission of water abstraction data for those metering points.
Terminology
Water abstraction refers to abstracting water from a source body of water.
Water meter refers to the physical device responsible for measuring the rate and volume of water taken.
Metering point refers to the location where Water Abstraction is measured, using a meter and its relationship to the consents which allow the abstraction.
Water Abstraction Point (WAP) refers to the location where water is abstracted.
Workflow
There are two main tasks for the API:
The setup task of creating metering points, used to link abstraction data to a WAP / consent pair that abstraction should be counted against
The ongoing task of submitting water abstraction data for a metering point
In addition, there are methods available for interrogation of data that has previously been created.
Endpoints
POST /metering-point
All water abstraction data must be submitted for a metering point. A metering point represents the place where a water meter is measuring the water being abstracted for a WAP on a consent (or set of WAPs and consents for complex scenarios). This is modelled independently of the water meter itself, as a water meter may be replaced while the metering point remains the same.
Metering points need to be created prior to use, by sending a POST request to the /metering-point endpoint.
Creating the metering point includes a list of WAP / consent pairs that water abstraction from the metering point will be counted against, and a name used to refer to the point. Optionally, creation of the metering point may provide some metadata including the geospatial coordinates of the metering point.
For registering non-standard setups (e.g. multiple consents/WAPs/meters) please see our FAQ below or contact us at waterusage@ecan.govt.nz.
The response to creating a metering point will be the return of a metering point key, which is an identifier for the point and will be used in subsequent submissions of water abstraction data.
Example using cURL
curl -X "POST" "https://apis.ecan.govt.nz/waterdata/water-abstraction/v1/metering-point"
-H 'Ocp-Apim-Subscription-Key: $Subscription-key'
--data $'{
"name": "Metering Point Name",
"description": "Metering Point Description",
"consentWaps": [
{ "consentNumber": "C000000", "wap": "A00/0000" }
]
}'
For this example, a response like below would be expected. Note, this request would actually fail because the WAP/consent combination does not exist.
{ "meteringPointKey": "00000000-0000-0000-0000-000000001234" }
Existing metering points that have the same WAP
If multiple metering points exist for the same WAP, and you've previously been sending us data for these sites via FTP, then we also need to know the existing meter number. There is an optional parameter available on the post /metering-point for this, called hilltopMeterNumber. You only need to provide this if the meter number is not 1.
E.g. If you have the following metering points with the same WAP AB12/123-M1 and AB12/123-M2 and you have previously sent data for these sites to us.
Example using cURL
For AB12/123-M1 the metering-point post will look like this. (Note, the hilltopMeterNumber is not required here, as the meter number is 1):
curl -X "POST" "https://apis.ecan.govt.nz/waterdata/water-abstraction/v1/metering-point"
-H 'Ocp-Apim-Subscription-Key: $Subscription-key'
--data $'{
"name": "Metering Point Name",
"description": "Metering Point with same WAP 1",
"consentWaps": [
{ "consentNumber": "C111111", "wap": "AB12/123" }
]
}'
For AB12/123-M2 the metering-point post will look like this:
curl -X "POST" "https://apis.ecan.govt.nz/waterdata/water-abstraction/v1/metering-point"
-H 'Ocp-Apim-Subscription-Key: $Subscription-key'
--data $'{
"name": "Metering Point Name 2",
"description": "Metering Point with same WAP 2",
"consentWaps": [
{ "consentNumber": "C111111", "wap": "AB12/123" }
] ,"hilltopMeterNumber": 2
}'
POST /metering-point/{meteringPointKey}/waterabstraction
Actual submission of abstraction data is done against the /waterabstraction resource which is a child of metering point. Sending a POST to this endpoint will create a record of water abstraction.
The request body includes details about what type of unit the abstraction is in, as well as a list of measurements which include the value and the time range the measurement represents. The unit types which are accepted are:
Cumulative m3: Abstraction data is represented as an ever-increasing number. It is measured in volume (cubic metres) and often referred to as water meter or meter reading i.e. the counter on a metering device.
Incremental m3: Abstraction data represents the volume of abstracted water between timestamps i.e. the amount of water abstracted in cubic metres for that interval.
Instantaneous L/s: Abstraction data in form of an instantaneous value can be extrapolated out to provide a m3 reading.
We accept data and time expression time formats according to ISO 8601. For example, YYYY-MM-DDThh:mm:ss+hh:mm
i.e. values "2022-01-08T04:05:06+13:00". If you do not specify a timezone, then it will default to UTC. Please ensure you are coding to deal with daylight saving changes when sending data in NZST/NZDT.
The response to submitting a water abstraction data return a receipt identifier, which can be used to retrieve the submitted water abstraction data.
Example using cURL
This is a request which includes 2 hours of data at 15 minute intervals.
curl -X "POST" "https://apis.ecan.govt.nz/waterdata/water-abstraction/v1/metering-point/00000000-0000-0000-0000-000000001234/waterabstraction"
-H 'Ocp-Apim-Subscription-Key: $Subscription-key'
--data $'{
"unitOfMeasure": "Incremental m3",
"interval": 15,
"measurements": [
{ "measurementTime": "2021-01-01T00:00:00+13:00", "measurementValue": 100, "previousMeasurementTime": "2020-12-31T23:45:00+13:00" },
{ "measurementTime": "2021-01-01T00:15:00+13:00", "measurementValue": 112, "previousMeasurementTime": "2021-01-01T00:00:00+13:00" },
{ "measurementTime": "2021-01-01T00:30:00+13:00", "measurementValue": 123, "previousMeasurementTime": "2021-01-01T00:15:00+13:00" },
{ "measurementTime": "2021-01-01T00:45:00+13:00", "measurementValue": 52, "previousMeasurementTime": "2021-01-01T00:30:00+13:00" },
{ "measurementTime": "2021-01-01T01:00:00+13:00", "measurementValue": 98, "previousMeasurementTime": "2021-01-01T00:45:00+13:00" },
{ "measurementTime": "2021-01-01T01:15:00+13:00", "measurementValue": 45, "previousMeasurementTime": "2021-01-01T01:00:00+13:00" },
{ "measurementTime": "2021-01-01T01:30:00+13:00", "measurementValue": 70, "previousMeasurementTime": "2021-01-01T01:15:00+13:00" },
{ "measurementTime": "2021-01-01T01:45:00+13:00", "measurementValue": 122, "previousMeasurementTime": "2021-01-01T01:30:00+13:00" },
{ "measurementTime": "2021-01-01T02:00:00+13:00", "measurementValue": 145, "previousMeasurementTime": "2021-01-01T01:45:00+13:00" }
]
}'
For the request above, a response like this would be expected. The receiptId can be used in get requests to identify.
{ "receiptId": "00000000-0000-0000-0000-000000004567" }
Frequently Asked Questions
General
Why should we make a change right now, when the existing FTP system still works?
The current FTP system has many quality and reliability issues. The move to a modern API-based solution is long overdue, and will provide advantages for all sides.
When will the FTP servers be turned off?
There is no specific date for this yet, but it will be clearly communicated closer to the time. Currently, the Water Abstraction API is still being developed to cater for all consenting scenarios and business tasks. Once development is further along, and there has been enough time to allow all data providers to build integrations with the system, the FTP servers will be decommissioned.
What information and documentation is available to help me build an integration with the new API?
For a general overview of how the API works, please see the documentation above, or once registered read the API Specifications under the product page. If you would like to start work on an integration, register an account on our Sandbox Developer Data Portal. Once you’ve created an account, send an email to developers@ecan.govt.nz with your details, and we will add you into our service provider security group, which will enable you to see the Water Abstraction API as a product in the sandbox.
Can I use a service account i.e. ‘admin@serviceprovider.co.nz' to register as a user and send data?
If you have a suitable service account available, the use of this would be preferable over setting up accounts for individual users. Please ensure access keys and passwords are treated as confidential information, and use appropriate systems and processes for security purposes according to our terms and conditions.
Can an external company develop an integration on our behalf?
Yes, an external company can develop the integration and can register on the sandbox environment to build and test a solution. However, it is important that the account that is registered on the production environment is owned by the party responsible for sending the data. In the situation where a consent holder signs a service agreement with a data provider, the data provider is responsible for sending the data in daily and ensuring they or their client can resolve any issues should they arise. Where an external party develops the solution but has no ongoing service arrangement with the consent holder, the production environment account must be held by the consent holder.
Technical
Why do I have to register a metering point before being able to send in abstraction data for that point?
By creating a metering point, we have explicit information about which consent particular water abstraction data is for. By making this a two-step process, we ensure things are set up correctly before any abstraction data starts being sent. The benefits of doing this are that issues are easier to resolve, we establish who is delivering service for which consents, we know who to follow up with in case of missing or corrupt data, and it helps ensure a smooth hand-over if a consent holder changes service providers.
Is it possible to register multiple metering points for one WAP/consent pair?
Yes this is possible. Every metering point registration should link to one data stream e.g. two meters can exist for the same WAP/consent pair if the pipe splits before the measurements take place. Multiple metering points are not intended for serial meters or parent and child meters. A distinct name/description for each endpoint is necessary, and GPS coordinates would also be helpful. When in doubt what to register, please contact us at waterusage@ecan.govt.nz.
Do I have to do a POST request for each measurement?
No, you can include many measurements in each POST of data. The expectation is that it’s 15-minute data delivered for each site daily, in accordance with the legislation. In the documentation for the API, the JSON message has an array of measurements to allow sending many in a request.
Are there restrictions on the amount of data that can be sent in an API call?
A years’ worth of 15-minute interval data (36,000 measurement values) is the maximum the API will accept in a single request.
Are we sending data in as URL parameters?
The POST URLs do include the identifier of the metering point in the path of them, following REST style. But the actual data being posted goes in the request body. You can see this in the documentation as the “body” listed under the POST requests.
What will you do for error or NaN or null data, that is something has gone wrong and I have missing data?
We’re working on a method for service providers to inform us that something has gone wrong and that there won’t be any data for a time period. The current service is for communicating good data only. So, in the case of a site being down or misbehaving this would just be missing data at the moment. Sending a measurement value of Null or NaN would be rejected by the API as that is not valid according to the JSON schema.
What checks are performed on the data?
The API validates the submitted water abstraction data with a couple of checks. We may add, remove or change checks in the future, but as a baseline they include:
A maximum of 36,000 measurement values are allowed in a single request (representing 1 years’ worth of 15-minute interval data)
The measurement value must be equal to or greater than 0. (Note: In the future we will allow negative values in certain circumstances, by exception).
The measurement timestamp must be between the year 2000 and now. Future dates will be rejected. Note, all submitted data is treated as UTC unless otherwise specified.
The interval between measurements must be between 1 and 1440 minutes.
What happens if we re-send the same data?
This is another use case that is on our roadmap, but we haven’t fully committed to a solution yet. At the moment, you can re-send the data. Regardless of whether the data was missing or you’re overwriting the previous data, we will store every submission that is made, so will have a full history of all versions of the data submitted to us.
What API payload format can we send the data in?
The Water Abstraction API only supports data sent via HTTPS in JSON format.
What is the purpose of the previousMeasurementTime when posting MeteringPoint data?
The previousMeasurementTime should be the timestamp of the most recent measurement recorded, i.e. just before the current measurement. For example, if there is a gap in the data (in which case the interval is longer than 15 minutes) then previousMeasurementTime should reference the measurement before the gap. This allows accurate calculation of the actual water abstraction over the stated period of time, and to verify the data submission and perform gap detection.
If this is the first time data is sent to ECan for this site, please use ‘measurementTime’ minus the interval length for previousMeasurementTime.
If you’re making a submission to fill a gap in the existing data, include the earliest measurement after the gap and link it up using the previousMeasurementTime parameter. See the diagrams below for a visual explanation.
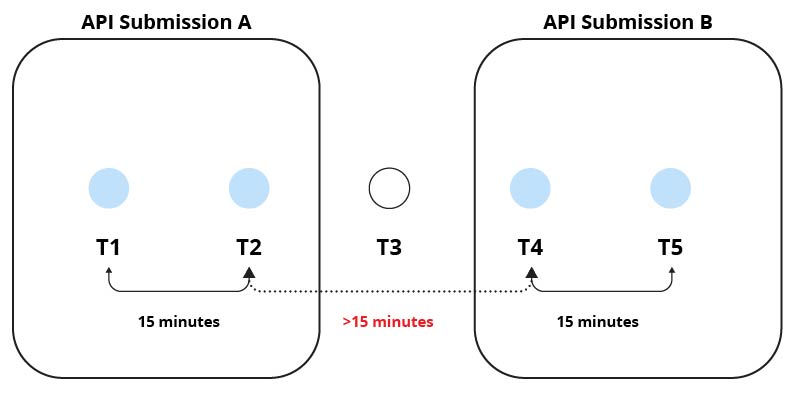
Diagram 1: Data submission with gap. In this scenario we’ve received data submissions which are separated by an interval of more than 15 minutes. The previousMeasurementTime links T4 and T2.
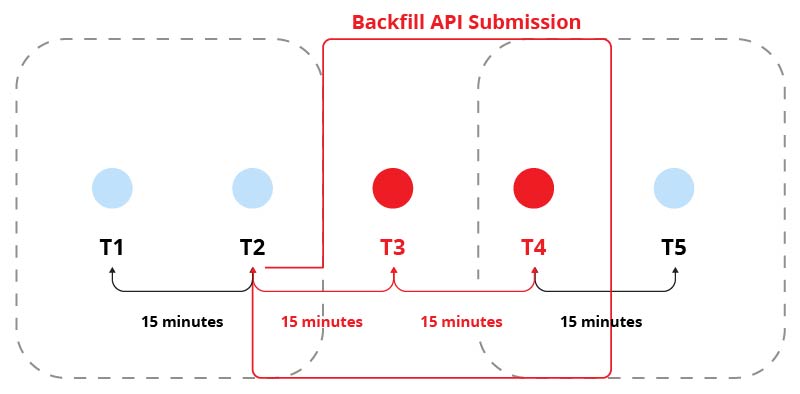
Diagram 2: Data submission filling gap. When sending the missing data to fill the gap, ensure the previousMeasurementTime parameter links the beginning and end of the new data with the existing submissions.
Finding more information
How can I look up a list of all consent numbers (CRC) and associated WAPs?
A list is available at https://data.ecan.govt.nz/Catalogue/Method?MethodId=86#tab-data. Note, the data has a 24-hr time lag. The preview only shows 50 rows, but if you click to download the data, you will get more.
How can I look up the WAPs for a single consent number?
This is available here: https://data.ecan.govt.nz/Catalogue/Method?MethodId=83#tab-data
How can I look up the consent number for a single WAP?
You can search by WAP here: https://data.ecan.govt.nz/Catalogue/Method?MethodId=81#tab-data
How can I look up consent details?
You can look up consent details manually via our Consent Search at https://www.ecan.govt.nz/data/consent-search/. To programmatically retrieve these details, there are a number of methods available: https://data.ecan.govt.nz/Catalogue/Search?CollectionId=1
How will I know when a consent number changes?
This is something we are looking into. We will either provide functionality to notify you of consent renewals proactively, or a means of allowing you to check for updates.
How can I look up a list of all Metering Points I've registered so far?
An endpoint is available to accomplish this, called GetMeteringPointCollection. You can find more details on the API specs page.
How can I look up the timestamp of the most recent Water Abstraction measurement supplied to a Metering Point?
An endpoint is available to accomplish this, called GetMeteringPointStatusQuery. You can find more details on the API specs page.
Roadmap
This is an evolving API, supported by Environment Canterbury developers. Feedback from our users helps to guide the features which are prioritised and added to our roadmap of changes. Below (in no particular order) are updates planned for the future:
Allowing for the resubmission of data
Allowing updates to metering points
Support
For any support queries, please contact the ECan developer team at developers@ecan.govt.nz