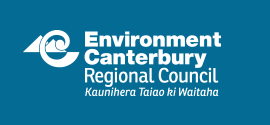
How do I use GraphQL APIs?
GraphQL is inherently simple, and works just fine without any specialised tooling. Let’s demonstrate this by showing several simple ways of calling a GraphQL API over HTTP.
Calling an API from the command line using cURL
Let's start by using cURL, which is of the most popular tools for accessing HTTP endpoints from the command line. The following command can be used to fetch GraphQL data from one of our APIs, the Environmental Observations API.
SUBSCRIPTION_KEY=Your_Subscription_Key
curl -X POST https://apis.ecan.govt.nz/waterdata/observations/graphql \
-H "Ocp-Apim-Subscription-Key: ${SUBSCRIPTION_KEY}" \
-H "Content-Type: application/json" \
--data @- --compressed <<EOF
{
"query": "{
getObservations {
locationId
name
type
unit
observations(filter: {start: \"2021-09-23 10:00:00\", end: \"2021-09-23 11:00:00\"}) {
timestamp
value
qualityCode
}
}
}"
}
EOF
The command above specifies following:
POST
HTTP verb. The standard way of retrieving data from GraphQL endpoints is by using aPOST
request to specify the query to be executed. We are using the cURL-X
option to achieve this.Content-Type
of application/json because we’re specifying the query as part of a JSON object.Ocp-Apim-Subscription-Key
header to identify the caller. For more information about subscription keys please follow this link.The data sent, which is a JSON document containing GraphQL query. In this example we are retrieving one hour of observations. Response should contain multiple observation lists. For each observation list, we want to retrieve locationId, name, type and unit. For each observation, we want a timestamp, value and quality code returned. Users can easily control which fields they want to receive in the response, by modifying
--data
parameter.--compressed
option because we are compressing responses by default to improve API performance. Passing--compressed
option informs cURL to convert the compressed binary response back to plain text.
Calling an API from JavaScript code using fetch
You should be able to make GraphQL requests in any programming language of your choice, but one of the most popular methods is calling APIs using JavaScript. The following code snippet uses fetch
, the new standard for getting HTTP responses using promises, to get the same dataset as the examples above. This will work in all modern browsers with no libraries. To make it work in some browsers (i.e. Internet Explorer) and Node, import isomorphic-fetch
library.
require('isomorphic-fetch');
fetch('https://apis.ecan.govt.nz/waterdata/observations/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Ocp-Apim-Subscription-Key': 'Your_Subscription_Key'
},
body: JSON.stringify({ query: `
query {
getObservations {
locationId
name
type
unit
observations(filter: { start: "2021-09-23 10:00:00", end: "2021-09-23 11:00:00" }) {
timestamp
value
qualityCode
}
}
}`
}),
})
.then(res => res.json())
.then(res => console.log(res.data));
References
This article by @stemmlerjs is another great resource for learning about interacting with GraphQL APIs.
What is GraphQL?
The description below is an extract from the GraphQL website, which is a good resource for learning more about GraphQL.
GraphQL is a query language for our APIs, and a runtime for fulfilling those queries with our existing data. GraphQL provides a complete and understandable description of the data in the APIs, and gives clients the power to ask for exactly what they need. While typical REST APIs usually require loading data from multiple URLs, GraphQL APIs get all the data your app needs in a single request from a central location. When using GraphQL APIs, you can access the full capabilities of our data from a single endpoint.
GraphQL APIs are organised in terms of types and fields, not endpoints. GraphQL uses types to ensure apps only ask for what’s possible and provide clear and helpful errors. Apps can use types to avoid writing manual parsing code.